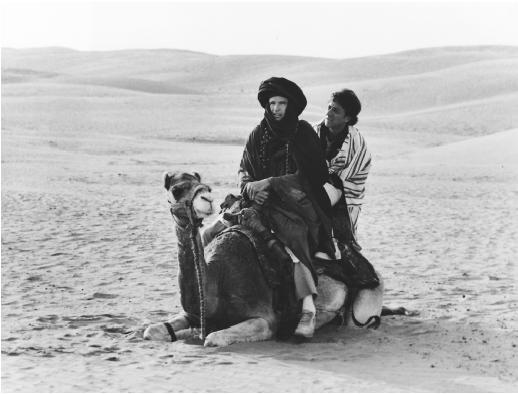
I'm happy to report the framerate for
Weapon of Choice on the Xbox 360 is playable! It's still not 'great' but it is 'okay'. I know that's not the best report but when the framerate made the game unenjoyable just a week ago, I'm feeling pretty good about it. And on a decent computer, the framerate is smoking (often around 80 fps)!
There were a few changes that yielded some large improvements. The first was to 'batch' the processing of the vertices. All of the images of the trees, monsters, etc are created using two triangles stuck together (a 'quad'). While this is like sprites in most games, these quads are slightly more complicated. They are
skinned to their vertices in a similar way to a
full 3D model in other games; because of this I can't use the built-in SpriteBatch system in XNA. Before the optimization I was simply rendering each quad. With the batching, the quads with the same texture are grouped together in a bigger list. An interesting optimization point is that around 50 quads gives the best results, while a larger list of 200 quads was faster than no batching, but not as fast as 50 quads. There's probably a lot of reasons for this, but
NProf suggests that it has to do with spending more time copying the larger vertex buffer needed in the 200 quad version. The framerate jumped to around 15% faster after the batching.
The second change that pushed the framerate even more was the use of
DXT compression for the textures. In the Content Pipeline in XNA all textures have an option to use this compression. The interesting thing is the default is 'Color' not DXT
(these are in the properties of the texture, under Content Processor, Texture format). I can't visibly tell a difference when using DXT compression, and the framerate increases by about 20% after this!
I found
this entry in Shawn Hargreaves' blog very useful for GPU optimization.
The last change I made was to actually shrink the biggest textures used. At their biggest some textures were around 1000x1000. I've shrunk them down to more like 600x600. In my particular case I think it helped supplement the framerate with the previous changes in effect by reducing the texture fetching time.
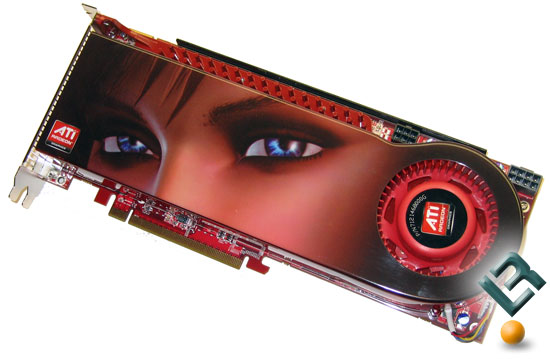
Currently I'm running into CPU slowdown on the Xbox and am exploring custom
shaders for faster drawing and multi-threading for the game object updates. Hopefully these get me the last boost for a great framerate on the Xbox!